Preference Center Integration Guide
The Preference Center in Optimove’s Web SDK allows users to manage their communication preferences for various topics, such as email, SMS, and push notifications. This enables personalized, compliant communication (e.g., for GDPR or marketing opt-ins). This guide covers implementing the Preference Center UI, managing preferences programmatically, and troubleshooting common issues. It assumes you’ve already set up the Web SDK; for setup instructions, see the Web SDK Integration.
Prerequisites
- Preference Center configured in Optimove.
- Web SDK initialized with a valid configuration token (see Web SDK Integration).
- Customer ID set for identified users.
Note: Ensure your SDK is properly initialized before implementing the Preference Center.
Implementation Types
The Preference Center offers two UI implementations:
Standard Topic-Based UI
A list-based interface where topics are toggleable options with global channel settings.
optimoveSDK.API.preferenceCenter.showUi();
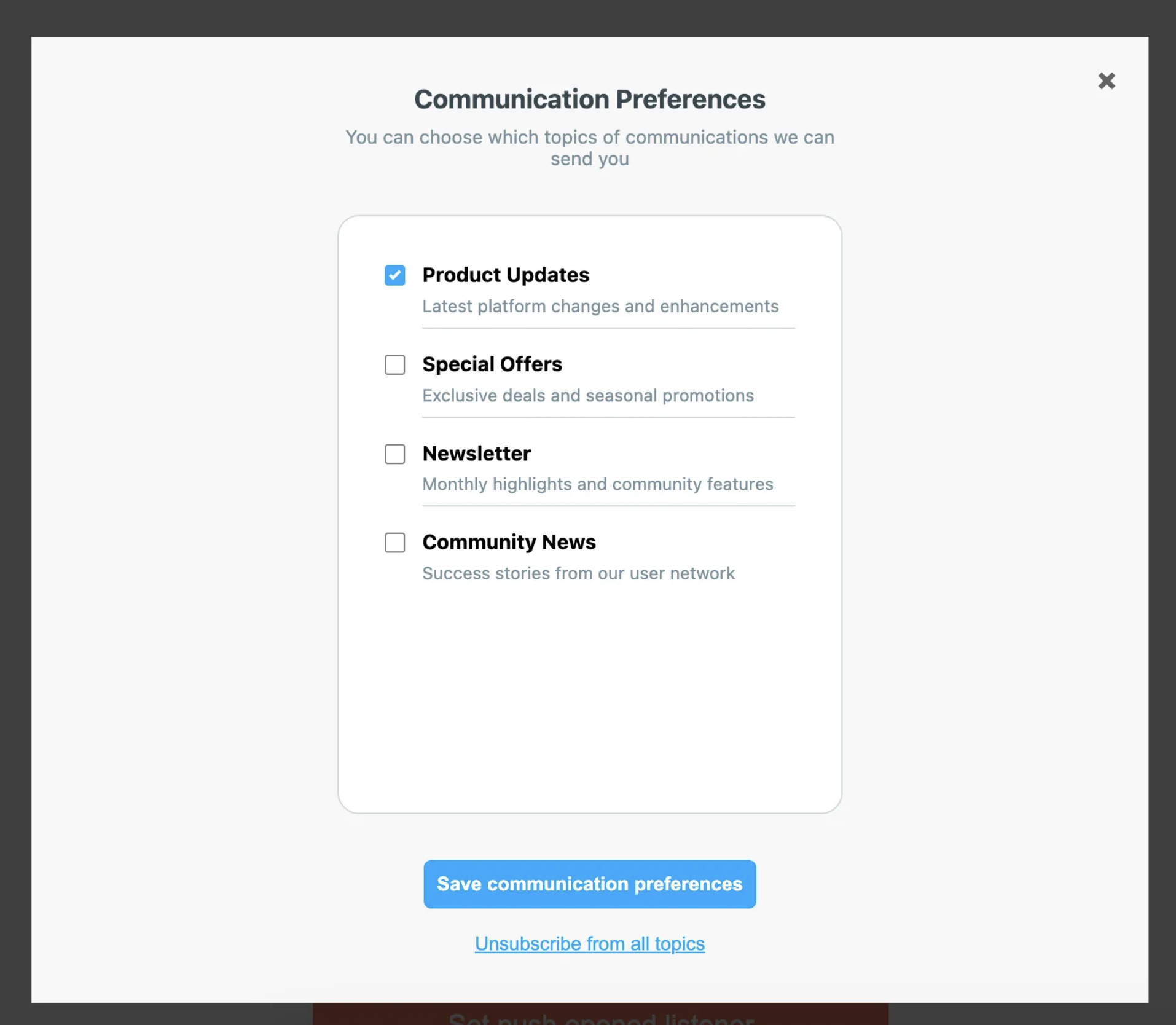
Figure 1: Standard Topic-Based UI for the Preference Center.
Advanced Channel-Topic Matrix UI
A matrix-style interface showing topics and channels, allowing channel-topic level customization.
optimoveSDK.API.preferenceCenter.showUi(1);
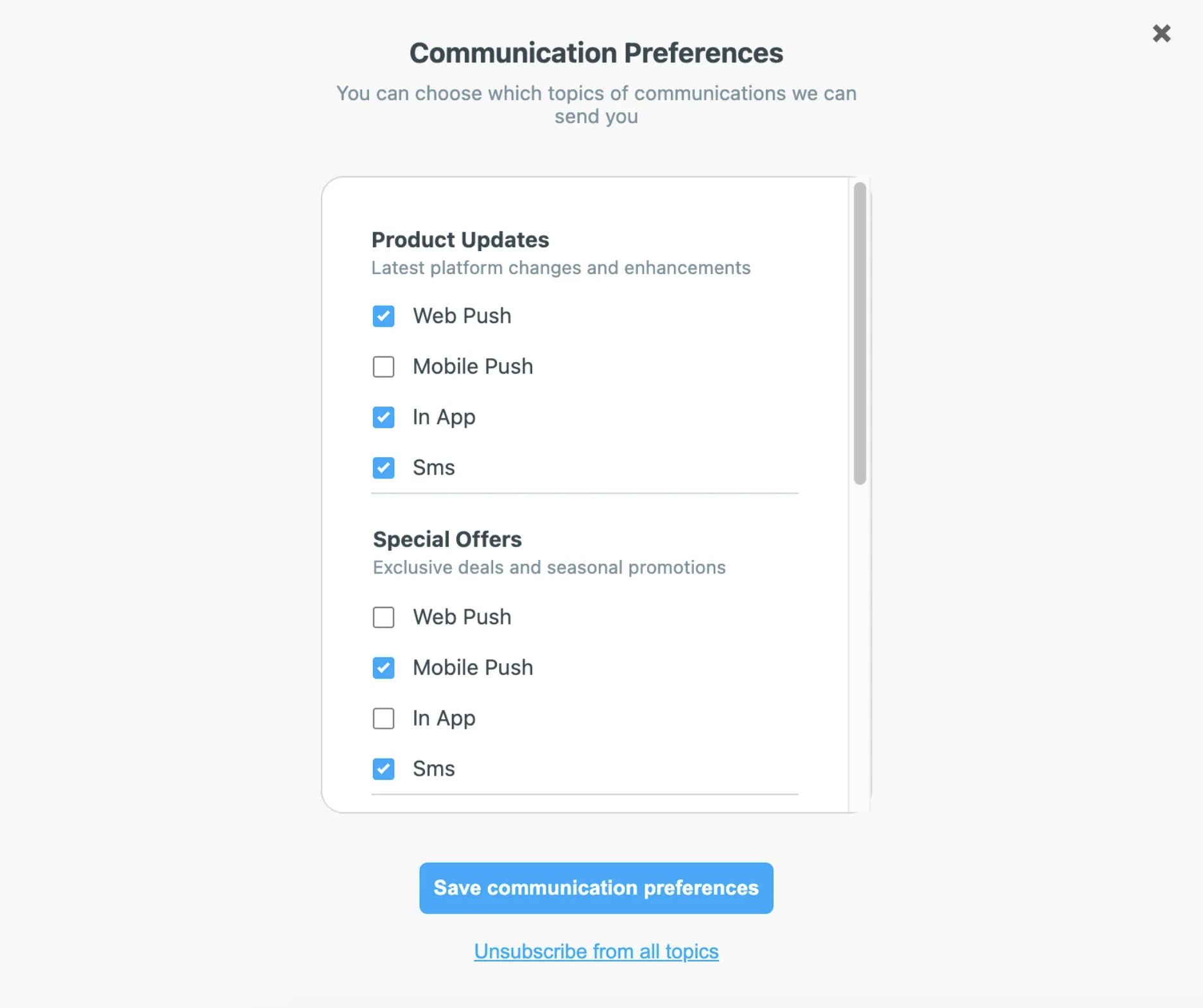
Figure 2: Advanced Channel-Topic Matrix UI on small screens.
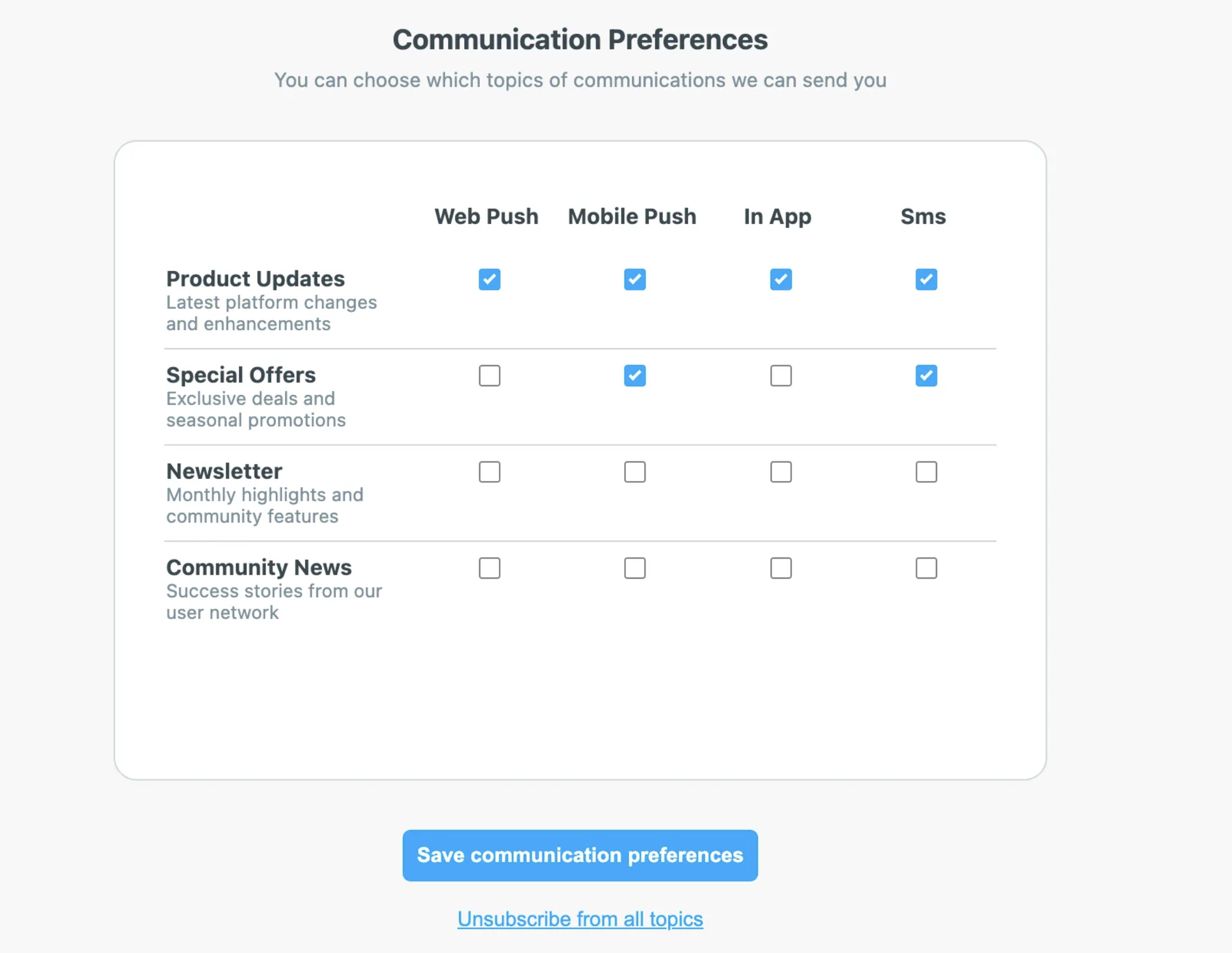
Figure 3: Advanced Channel-Topic Matrix UI on larger screens.
Getting Customer Preferences
Retrieve a customer’s communication preferences using the getPreferences
method:
optimoveSDK.API.preferenceCenter.getPreferences(customerId)
.then(preferences => {
console.log('Customer preferences:', preferences);
})
.catch(error => {
console.error('Error fetching preferences:', error);
});
Response Structure
The method returns a Promise resolving to a Preferences
object:
{
customerPreferences: [
{
id: string,
name: string,
description: string,
subscribedChannels: [int] // Channels the customer is subscribed to for this topic
}
// Additional topics...
],
configuredChannels: [int] // Array of all channels configured for the brand group
}
Channel ID Reference
- Email: 15
- Mobile Push: 489
- Web Push: 490
- SMS: 493
- In-App: 427
- WhatsApp: 498
- Inbox: 495
Use these IDs to interpret subscribedChannels
and configuredChannels
or specify channels in preference updates.
Updating Customer Preferences
Update preferences using the setCustomerPreferences
method:
const updates = [
{
topicId: "topic-123",
subscribedChannels: [15, 493]
},
{
topicId: "topic-456",
subscribedChannels: [489]
}
];
optimoveSDK.API.preferenceCenter.setCustomerPreferences(customerId, updates)
.then(() => {
console.log('Preferences updated successfully');
})
.catch(error => {
console.error('Error updating preferences:', error);
});
Update Format
The updates
parameter is an array of PreferenceUpdate
objects:
{
topicId: string,
subscribedChannels: [int] // Array of channel IDs the customer wants to subscribe to
}

Figure 4: Workflow for updating customer preferences.
Example: Building a Custom Preference Interface
optimoveSDK.API.preferenceCenter.getPreferences(customerId)
.then(preferences => {
renderCustomPreferenceUI(preferences);
});
function handlePreferenceSubmit(formData) {
const updates = convertFormToUpdates(formData);
optimoveSDK.API.preferenceCenter.setCustomerPreferences(customerId, updates)
.then(() => {
showSuccessMessage();
})
.catch(error => {
showErrorMessage(error);
});
}
Use Case
Imagine you’re running an e-commerce website in the EU, where GDPR compliance requires explicit user consent for marketing communications. You implement the Preference Center to let users opt into SMS notifications for sales and email newsletters while opting out of promotional web push notifications. Using the Advanced Channel-Topic Matrix UI, users customize their preferences, and you retrieve these settings with getPreferences to ensure compliance before sending campaigns.
Troubleshooting
Common issues and solutions:
- Preference Center UI Not Displaying:
- Verify SDK initialization and customer ID.
- Test in a development environment before production deployment.
- Error Fetching Preferences:
- Check if the customerId is valid and matches an identified user.
- Ensure the Web SDK is initialized with a valid configuration token.
- Preference Updates Failing:
- Validate the updates array format (e.g., topicId must be a string, subscribedChannels must be an array of integers).
- Confirm the channel IDs match those in your Optimove instance.
- Inconsistent Preferences Across Channels:
- Ensure the customer ID is consistently set across all interactions.
- Avoid race conditions by waiting for getPreferences to resolve before calling setCustomerPreferences.
Further Reading
Updated 2 months ago